A stack pointer is a register in a computer’s CPU (Central Processing Unit) that stores the address of the topmost item on the stack. The stack is a data structure used in computer science that stores a collection of elements and provides two primary operations: push and pop.
The pop operation removes the topmost item from the stack. When a push operation is performed, the stack pointer is incremented to point to the new topmost item, and when a pop operation is performed, the stack pointer is decremented to point to the next item on the stack.
The stack pointer is used extensively in computer programming, particularly in low-level programming languages such as assembly language. It is used to manage the function call stack, which is a stack of function calls and their associated variables that are created and destroyed as a program runs.
In summary, the stack pointer is a register in the CPU that points to the topmost item on the stack, and it is used extensively in computer programming to manage the function call stack.
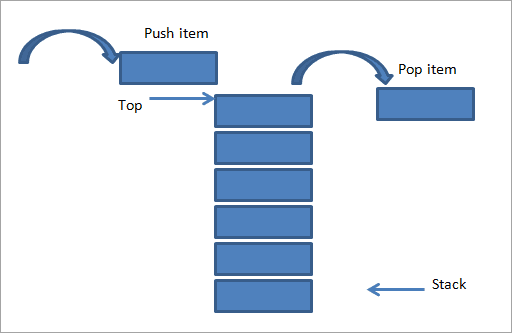
Types of Stack:
There are several types of stack data structures, each with its own characteristics and applications. Here are some of the most common types of stacks:
- Static Stack: A static stack is a fixed-size stack, meaning that the maximum number of elements that can be stored in the stack is predetermined. Once the stack is full, no more elements can be added. Static stacks are typically implemented using arrays.
- Dynamic Stack: A dynamic stack is a variable-size stack, meaning that the number of elements that can be stored in the stack can grow or shrink as needed. Dynamic stacks are typically implemented using linked lists.
- LIFO (Last-In-First-Out) Stack: A LIFO stack is the most common type of stack, where the last item added to the stack is the first one to be removed. This type of stack is used extensively in computer programming, particularly in function call stacks.
- FIFO (First-In-First-Out) Stack: A FIFO stack, also known as a queue, is a stack where the first item added to the stack is the first one to be removed. This type of stack is used in situations where data needs to be processed in the order it was received, such as message queues.
- Priority Stack: A priority stack is a stack where each item is assigned a priority value, and items with higher priority are given precedence over items with lower priority. This type of stack is used in scheduling and other applications where certain tasks need to be performed before others.
- Undo Stack: An undo stack is a special type of stack that is used in software applications to implement an undo feature. Each action performed by the user is added to the stack, and the user can then undo the last action by removing it from the stack.
In summary, there are several types of stack data structures, including static and dynamic stacks, LIFO and FIFO stacks, priority stacks, and undo stacks. Each type of stack has its own characteristics and applications, and choosing the right type of stack depends on the specific requirements of the problem being solved.
Register Stack:
A register stack is a type of stack that is implemented using the registers of a CPU (Central Processing Unit). In a register stack, the top of the stack is stored in a specific register, typically called the stack pointer register, and the other elements of the stack are stored in other registers.
The main advantage of using a register stack is speed. Since registers are part of the CPU, they can be accessed much faster than memory. This makes register stacks ideal for applications where speed is critical, such as real-time systems and embedded systems.
Register stacks are typically used in low-level programming languages such as assembly language, where the programmer has direct control over the hardware. However, they are not commonly used in higher-level programming languages such as C or Java, where the memory management is handled by the programming language runtime.
In summary, a register stack is a type of stack that is implemented using the registers of a CPU, providing fast access to the top of the stack. Register stacks are ideal for applications where speed is critical, but they are not commonly used in higher-level programming languages.
Memory Stack:
A memory stack, also known as a call stack or program stack, is a type of stack data structure used in computer programming to store information about the active subroutines (also known as functions or procedures) of a program.
When a subroutine is called, the program pushes information about the subroutine onto the stack, including the return address (i.e., the location in the program where the subroutine was called from) and any arguments passed to the subroutine. This creates a new stack frame on top of the stack.
As the subroutine executes, local variables and other information are stored in the stack frame. When the subroutine returns, the program pops the stack frame off the stack, restoring the previous stack frame and returning control to the calling subroutine.
The memory stack is an important component of program execution, as it allows the program to keep track of which subroutines are active and to return control to the appropriate location when a subroutine returns.
The memory stack is typically implemented in computer memory, either in the stack segment of the program’s memory space or in the heap. It is used in most programming languages, including high-level languages such as Java and Python, as well as low-level languages such as assembly language.
In summary, a memory stack is a type of stack data structure used in computer programming to store information about the active subroutines of a program. It is an important component of program execution, allowing the program to keep track of which subroutines are active and to return control to the appropriate location when a subroutine returns.
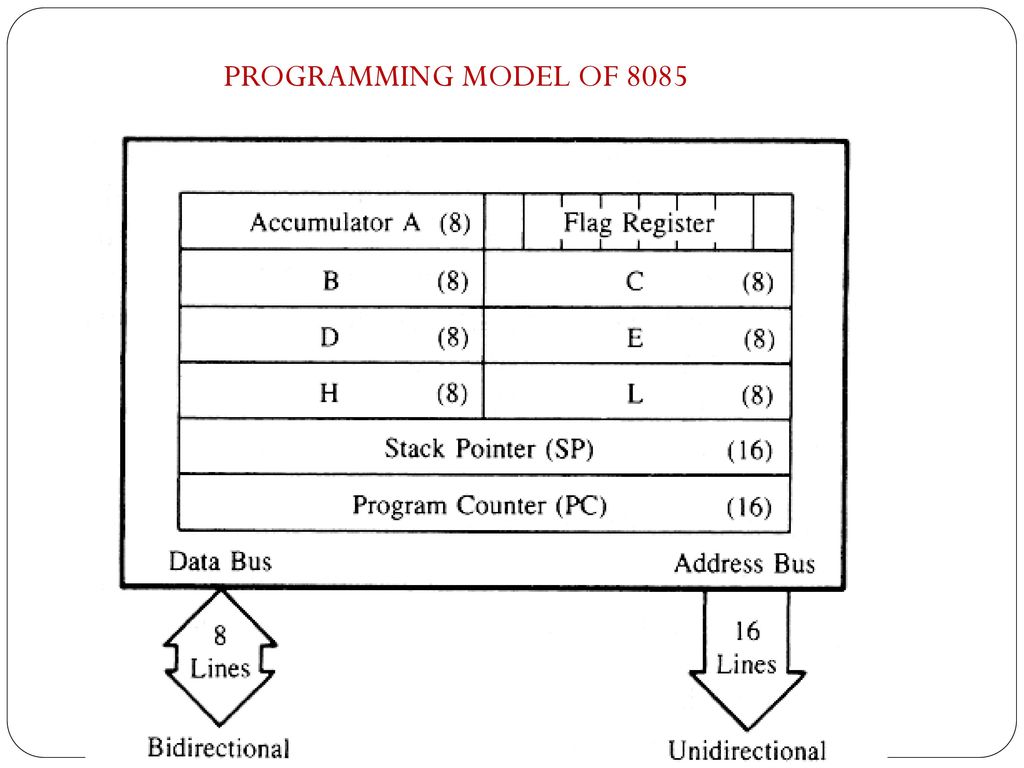
Stack/Stack Pointer in 8085 Microprocessor:
The 8085 microprocessor has a hardware stack, also known as the stack memory, which is a special region in the memory used to store data temporarily. The stack pointer register (SP) is used to keep track of the top of the stack. The SP points to the memory location where the next value will be pushed onto the stack.
Here are some key points related to the stack and stack pointer in the 8085 microprocessor:
- The stack memory is located in the memory space of the 8085 microprocessor. The size of the stack memory is 256 bytes and it starts at memory location 0x0100.
- The stack pointer (SP) is a 16-bit register that points to the top of the stack. Initially, the SP is set to 0xFF.
- The stack operations are performed using two instructions: PUSH and POP. PUSH is used to push a value onto the stack, while POP is used to remove a value from the top of the stack.
- When a value is pushed onto the stack, the value is stored in the memory location pointed to by the SP, and the SP is decremented by 1.
- When a value is popped from the stack, the value is retrieved from the memory location pointed to by the SP, and the SP is incremented by 1.
- The stack is a last-in-first-out (LIFO) data structure, which means that the last value pushed onto the stack is the first value to be popped.
- The stack can be used for various purposes in the 8085 microprocessor, such as storing return addresses, saving register values during subroutine calls, and passing parameters to subroutines.
In summary, the 8085 microprocessor has a hardware stack and a stack pointer register that is used to manage the stack operations. The stack can be used for various purposes, including storing return addresses, saving register values, and passing parameters to subroutines.
Basic Operations of Stack/Stack Pointer
The basic operations of the stack and stack pointer include the following:
- PUSH: This operation is used to insert data onto the stack. When a data item is pushed onto the stack, it is stored at the memory location pointed to by the stack pointer (SP) and the stack pointer is decremented by the size of the data item. For example, if the data item is one byte, the SP is decremented by 1.
- POP: This operation is used to remove data from the stack. When a data item is popped from the stack, the value at the memory location pointed to by the SP is retrieved and the SP is incremented by the size of the data item.
- TOP: This operation is used to retrieve the value at the top of the stack without removing it. The value at the memory location pointed to by the SP is returned.
- EMPTY: This operation is used to check if the stack is empty. The stack is considered empty if the stack pointer points to the beginning of the stack (i.e., the stack pointer has not been decremented).
- FULL: This operation is used to check if the stack is full. The stack is considered full if the stack pointer points to the end of the stack (i.e., the stack pointer has reached the maximum size of the stack).
These operations allow the stack to be used as a LIFO (Last-In-First-Out) data structure, where the last item pushed onto the stack is the first item to be popped off. The stack is commonly used in computer programming to store information such as function return addresses, local variables, and parameters passed to functions.